Why use image hover effects
As we evolve into a new age of digital interactivity, the need for engaging and interactive user interface designs has become increasingly important. Among the numerous techniques used to enhance user experience, image hover animations have emerged as a popular tool. By bringing static images to life when a user hovers over them, it adds a dynamic, captivating element to a webpage, resulting in increased engagement and interactivity.
In this article we show you how to achieve all these popular image hover effects with 2 methods:
Method 1: Manually with code using HTML, CSS and JS
Method 2: No code required, by using the PageGPT chat interface
Image Hover Tilt Effect
The image hover tilt effect adds depth and dimension to a webpage, engaging users with a playful, interactive twist on a static image.
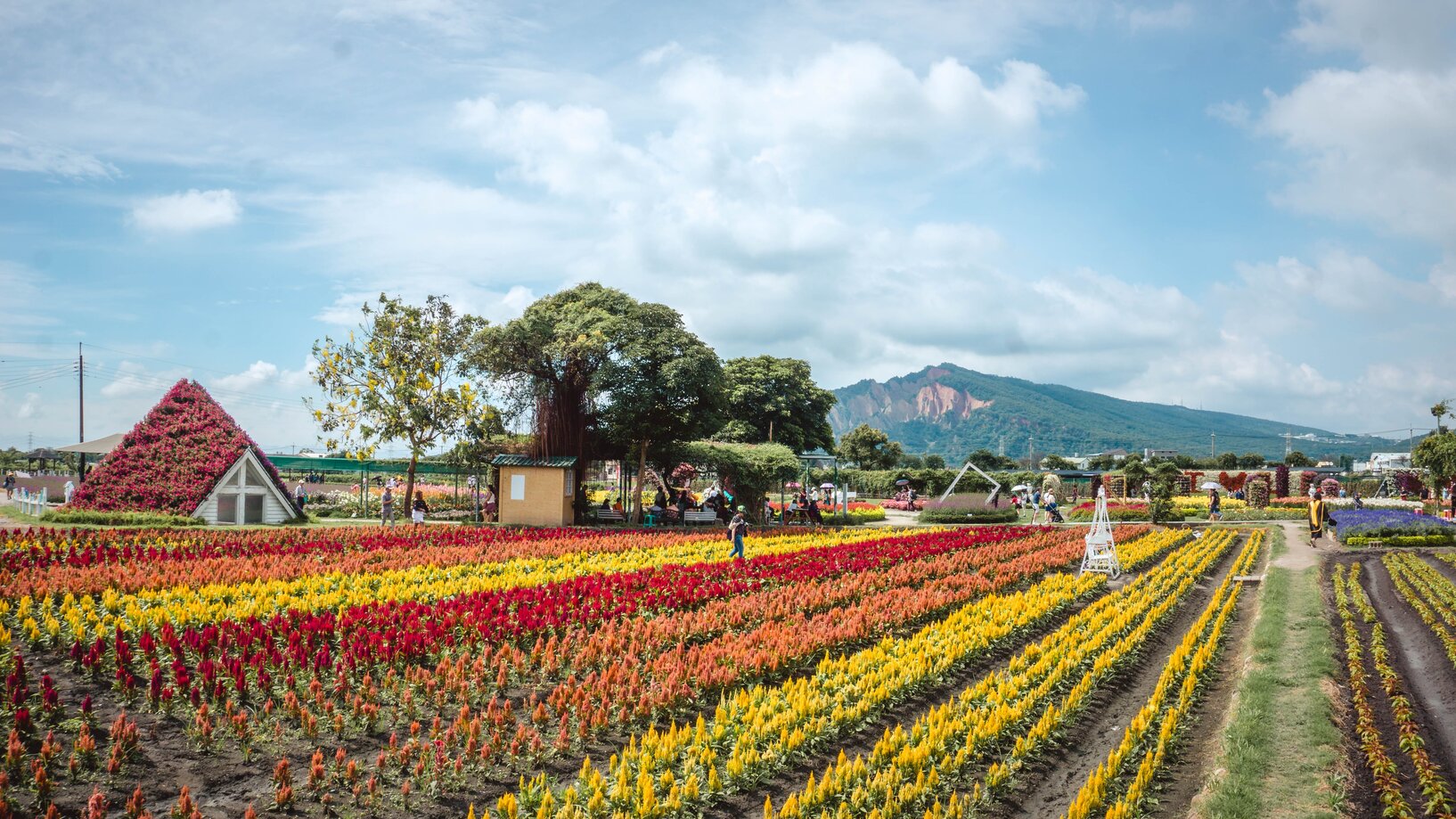
How to accomplish with code
This technique requires custom CSS and JS code. The JS code adds an event listener to determine the position of the cursor with respect to the center of the image.
We can then use this information to calculate the amount of rotation to add to the image. Here’s the code for the above example
<div class="hoverImageWrapper">
<img class="hoverImage" alt="" src="https://image.url" />
</div>
.hoverImage {
position: relative;
align-self: stretch;
height: 250px;
flex-shrink: 0;
object-fit: cover;
border-radius: 10px;
max-width: 100%;
}
window.onload = function() {
const imageElement = document.querySelector('.hoverImage');
if (imageElement) {
const handleMouseMove = (e) => {
let rect = imageElement.getBoundingClientRect();
let x = e.clientX - rect.left;
let y = e.clientY - rect.top;
let dx = (x - rect.width / 2) / (rect.width / 2);
let dy = (y - rect.height / 2) / (rect.height / 2);
imageElement.style.transform = `perspective(500px) rotateY(${dx * 5}deg) rotateX(${-dy * 5}deg)`;
};
const handleMouseLeave = () => {
imageElement.style.transform = "";
};
imageElement.addEventListener('mousemove', handleMouseMove);
imageElement.addEventListener('mouseleave', handleMouseLeave);
}
}
With PageGPT
Creating all sorts of image hover effects with PageGPT is easy. You simply have to select your image and enter your requirements into the chat box.
In this example we’re using the prompt: “add a hover effect where the image tilts in the direction of the cursor”
The first time the tilt was too slight, and the second time the tilt was too much, so we had to tweak it by chatting with it and giving instructions to make the tilt slightly less.
Hover Blur with Text Effect
Hover blur with text effect involves blurring an image on hover and simultaneously revealing text. This is great for revealing information related to the image when the user hovers over it.
How to accomplish with code
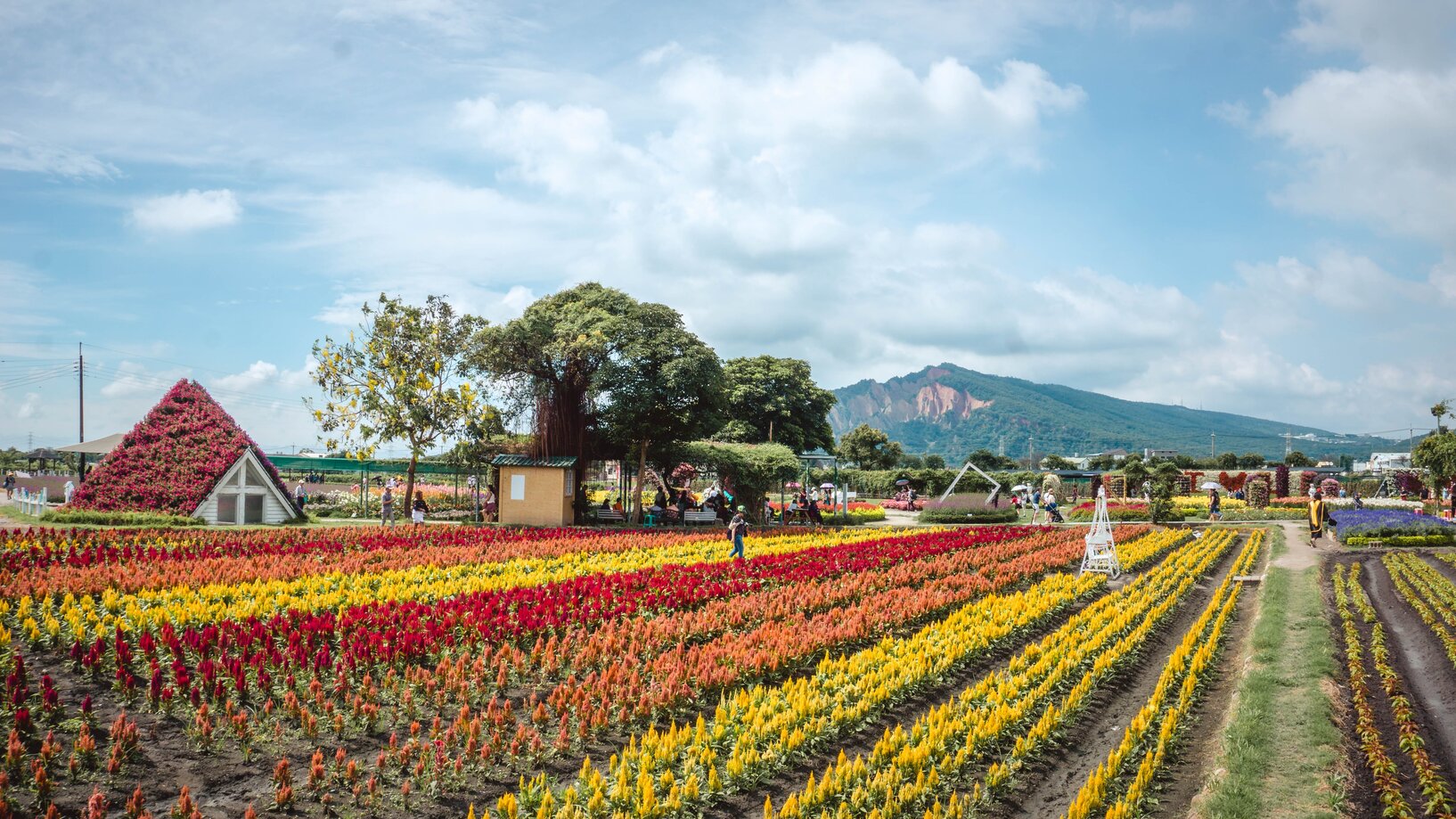
This effect can be achieved using CSS filters and transitions. We also make use of the ::after pseudo-element to add the text that we need.
<div class="hoverImageWrapper">
<img class="hoverImage" alt="" src="https://image.url" />
</div>
.hoverImageWrapper {
position: relative;
display: flex;
}
.hoverImage {
position: relative;
align-self: stretch;
height: auto;
flex-shrink: 0;
object-fit: cover;
border-radius: 10px;
max-width: 100%;
transition: filter 0.5s;
}
.hoverImageWrapper::after {
content: "Your Text Here";
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
color: white;
text-align: center;
display: flex;
justify-content: center;
align-items: center;
opacity: 0;
transition: opacity 0.5s;
pointer-events: none;
}
.hoverImageWrapper:hover .hoverImage {
filter: blur(8px);
}
.hoverImageWrapper:hover::after {
opacity: 1;
}
WITH PAGEGPT
Once again, we can simply type into the chat box to let PageGPT know what we’re looking for. In this scenario we’re using this prompt: “add a blur effect to this image on hover, with text in the middle saying ‘Coastal Photography’”
The first two times it didn’t work, so we simply clicked on “no” to let PageGPT know to try again, and we got it perfectly on it’s third go!
Image Zoom on Hover Effect
The image zoom on hover effect enlarges the image when the user hovers over it, providing a dynamic, magnifying effect that draws attention and increases engagement.
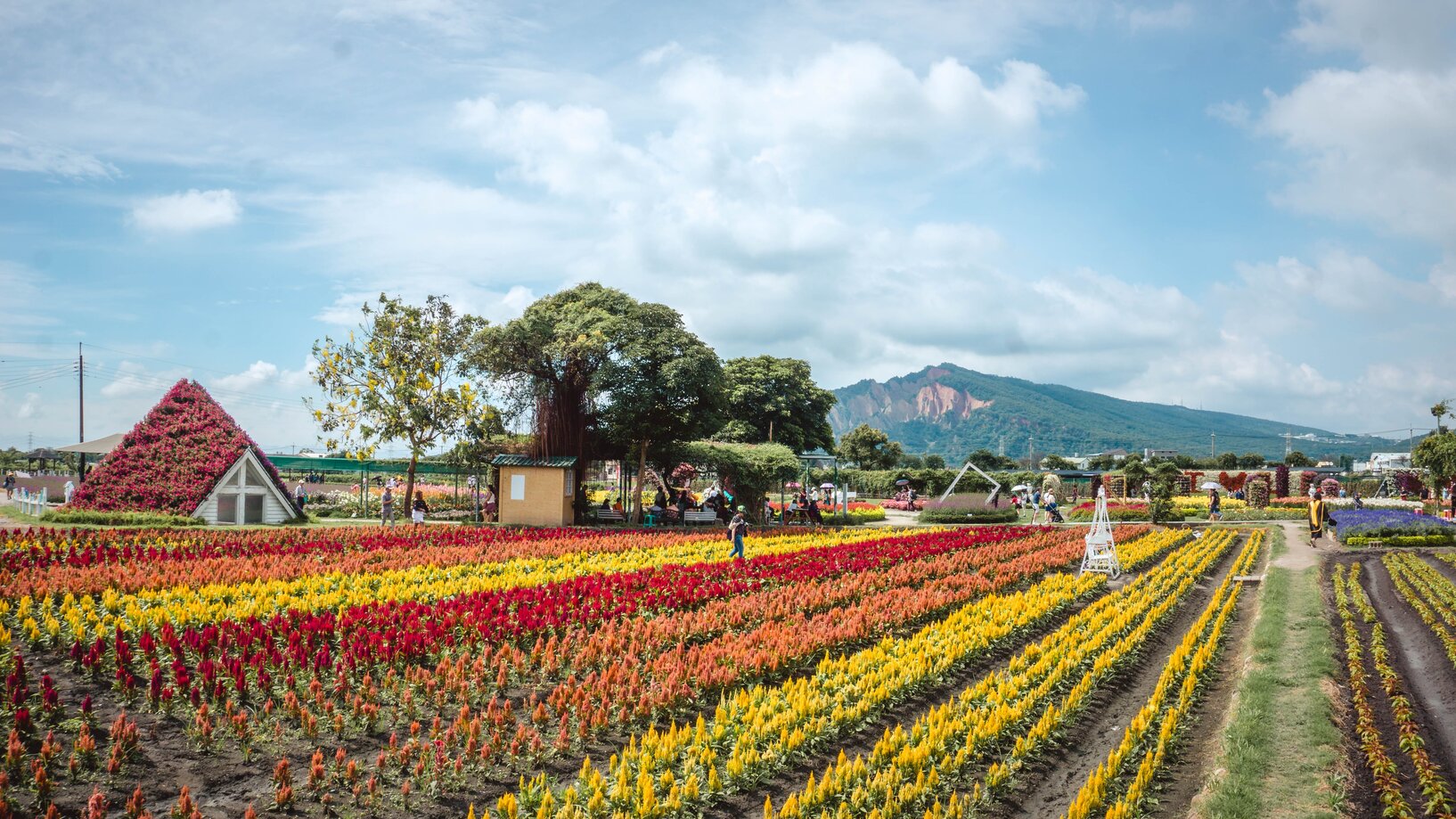
How to accomplish with code
This effect can be accomplished by using CSS transitions and transforms. We use the scale transform method to create the zoom in effect.
<div class="hoverImageWrapper">
<img class="hoverImage" alt="" src="https://image.url" />
</div>
.hoverImage {
position: relative;
align-self: stretch;
height: auto;
flex-shrink: 0;
object-fit: cover;
border-radius: 10px;
max-width: 100%;
transition: transform 0.5s ease-in-out;
}
.hoverImageWrapper:hover .hoverImage {
transform: scale(1.2);
}
With PAGEGPT
You guessed it, to do this with PageGPT is as simple as chatting. Here we used the prompt “make the image zoom in on hover” in order to achieve the effect.
It worked on the first and second try, but it wasn’t exactly what we was going for. In the third try, we got a beautiful ease in zoom effect that we wanted to achieve.
Hover Overlay with Text Effect
The hover overlay with text effect overlays an image with text when the user hovers over it. This can be used to provide additional information or interaction related to the image.
How to accomplish with code
This can be accomplished in a similar fashion to the blur effect that we had added earlier. This will require using the ::after pseudo-element as well.
Instead of adding the blur filter, we added a transparent dark background.
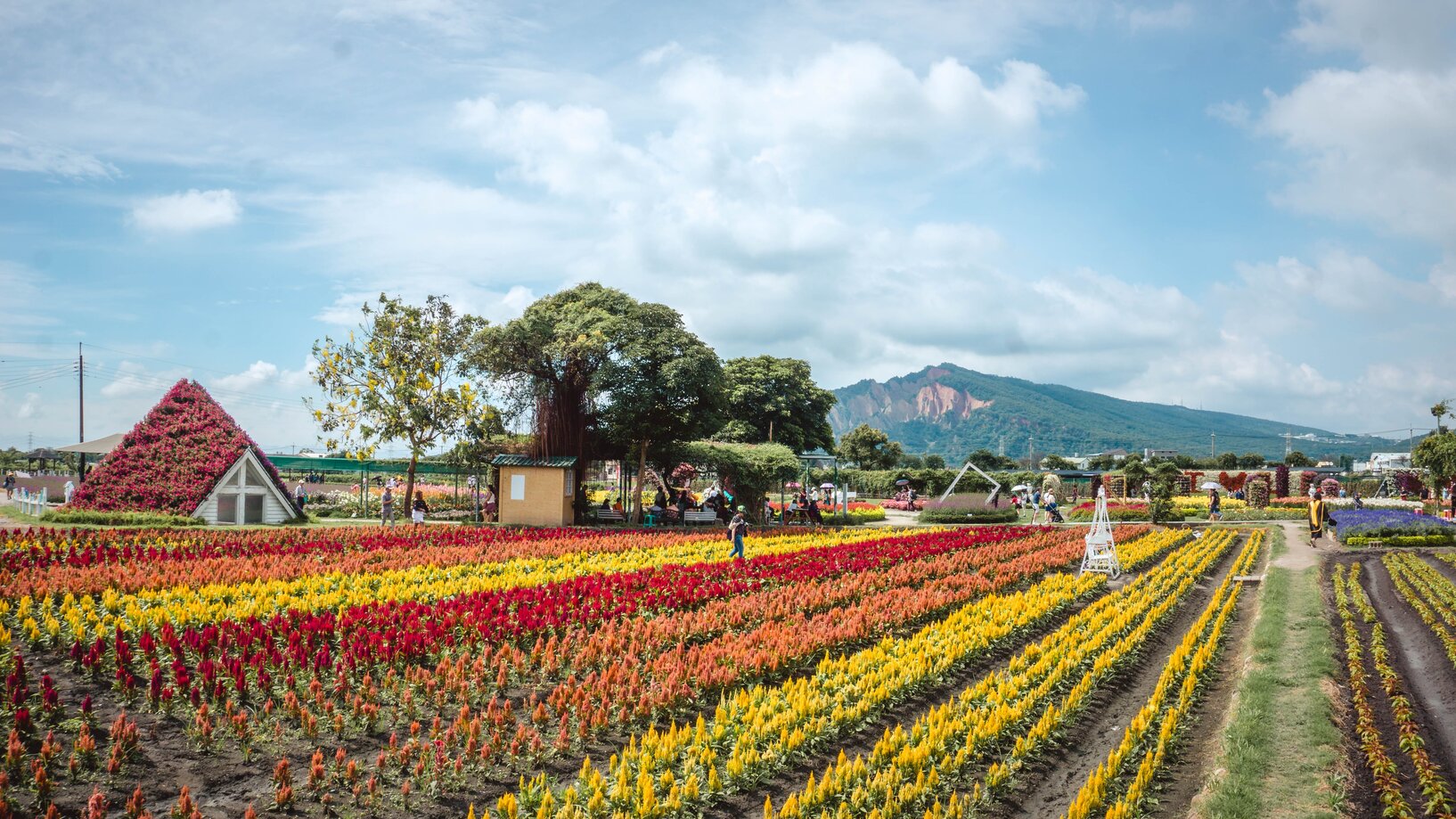
<div class="hoverImageWrapper">
<img class="hoverImage" alt="" src="https://image.url" />
</div>
.hoverImageWrapper {
position: relative;
display: flex;
}
.hoverImage {
position: relative;
align-self: stretch;
height: auto;
flex-shrink: 0;
object-fit: cover;
border-radius: 10px;
max-width: 100%;
transition: opacity 0.5s;
}
.hoverImageWrapper::after {
content: "Your Text Here";
border-radius: 10px;
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
color: white;
text-align: center;
display: flex;
justify-content: center;
align-items: center;
background: rgba(0, 0, 0, 0.7);
opacity: 0;
transition: opacity 0.5s;
pointer-events: none;
}
.hoverImageWrapper:hover .hoverImage {
opacity: 0.3;
}
.hoverImageWrapper:hover::after {
opacity: 1;
}
With PAgeGPT
To get this overlay effect in PageGPT, we just had to enter the following prompt into the chatbox, and viola – we have our hover overlay effect!
“When I hover over the image, i want a dark overlay with text in the middle saying ‘Coastal Photography’”
And just like that we got our overlay effect. Easy as pie!
Conclusion
In conclusion, image hover animations have shown to significantly enhance user interaction and engagement. By adding a new level of depth and interactivity, these effects can transform static web pages into dynamic experiences. Whether you’re using the tilt effect to create 3D interactions, blurring and showing text for added intrigue, zooming for detail, overlaying text for context, these tools can make a significant difference in your user interface design.
You can add them using the HTML,CSS and JS techniques above, or simply use PageGPT to add all the effects for you. On top of just image hover effects, you can chat to add all sorts of other effects like scroll animations, gradient text, parallax effects and more just by chatting with PageGPT.
Master all these techniques and see your user engagement soar!